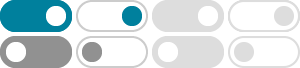
6.3. The Sequential Search — Problem Solving with Algorithms …
The Sequential Search¶ When data items are stored in a collection such as a list, we say that they have a linear or sequential relationship. Each data item is stored in a position relative to the others. In Python lists, these relative positions are the index values of the individual items.
Searching Algorithms - Linear and Binary Search (Python)
Jul 20, 2021 · Time complexity of Binary Search. The running time complexity for binary search is different for each scenario. The best-case time complexity is O(1) which means the element is located at the mid-pointer. The Average and Worst-Case time complexity is O(log n) which means the element to be found is located either on the left side or on the right ...
Binary Search – Algorithm and Time Complexity Explained
Jul 12, 2023 · You can always run a sequential search—scanning the array from the beginning to the end—on the array. But if the array is sorted, running the binary search algorithm is much more efficient. Let's learn how binary search works, its time complexity, and code a simple implementation in Python. How Does Linear Search Work?
The Sequential Search - bradfieldcs.com
Sequential search of a list of integers. The Python implementation for this algorithm is shown below. The function needs the list and the item we are looking for and returns a boolean value as to whether it is present.
Sequential and Binary search with Python | by Fell | Medium
Jan 3, 2024 · In an application that runs many times a day it could be crucial, but easily avoidable. The sequencial search has a time complexity of O (n), which means that the time is directly linked to...
Time Complexity in Python - Medium
Jul 27, 2020 · A linear or sequential search, is done when you inspect each item in a list one by one from one end to the other to find a match for what you are searching for.
Linear Search in Python - Know Program
The algorithm for linear or sequential search can be given as, def linearSearch(arr, key): for i in range(len(arr)): if (arr[i] == key): return i return -1. Time complexity of the above algorithm:- O(n) Best-case performance:- O(1).
Sequential Search Algorithm Time Complexity | Restackio
The time complexity of the sequential search algorithm is O(n), where n is the number of elements in the list. This means that in the worst-case scenario, the algorithm will need to check every element in the list.
Sequential and Binary Search in Python - GitHub Pages
When searching for a specific datum within the array, we are able to seqeuntially evaluate each item in the list, or array, to see if it matches the item we’re looking for. Using sequential_search, we simply move from item to item in the list, evaluating whether our search expression is …
Sequential Search or Linear Search in Python - Codez Up
In computer science, a linear search or sequential search is a method for finding an element within a list. It sequentially checks each element of the list until a match is found or the whole list has been searched.