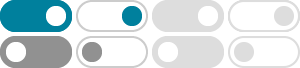
python - Sorting arrays in NumPy by column - Stack Overflow
May 13, 2010 · How do I sort a NumPy array by its nth column? For example, given: a = array([[9, 2, 3], [4, 5, 6], [7, 0, 5]]) I want to sort the rows of a by the second column to obtain: a...
NumPy Array Sorting | How to sort NumPy Array - GeeksforGeeks
Feb 1, 2024 · Sorting the NumPy array makes finding duplicate, maximum, and minimum elements easier. It is an essential operation of data manipulation, making it easier to work with data. In this tutorial, we have covered three methods on how to sort a array in NumPy i.e., sort(), argsort() and lexsort().
python - Efficiently sorting a numpy array in descending order?
Nov 18, 2014 · For short arrays I suggest using np.argsort() by finding the indices of the sorted negatived array, which is slightly faster than reversing the sorted array: a[np.argsort(-a)] is probably the best approach to any others on this page. No -1 step reversal and one less minus sign to think about. np.flip() and reversed indexed are basically the same.
numpy.sort — NumPy v2.2 Manual
numpy.sort# numpy. sort (a, axis =-1, kind = None, order = None, *, stable = None) [source] # Return a sorted copy of an array. Parameters: a array_like. Array to be sorted. axis int or None, optional. Axis along which to sort. If None, the array is flattened before sorting. The default is -1, which sorts along the last axis.
python - How to sort pandas dataframe in ascending order - Stack Overflow
sort_values() sorts the data in ascending order by default. You are interested in .sort_values(by='Date', ascending=False): import pandas as pd df = pd.DataFrame(range(10), columns=['Price']) df['Date'] = [pd.to_datetime(f"2021-02-{str(x).zfill(2)}") for x in range(1, 11)] df.sort_values(by=['Date'], ascending=False)
pandas.DataFrame.sort_values — pandas 2.2.3 documentation
pandas.DataFrame.sort_values# DataFrame. sort_values (by, *, axis = 0, ascending = True, inplace = False, kind = 'quicksort', na_position = 'last', ignore_index = False, key = None) [source] # Sort by the values along either axis. Parameters: by str …
How to Sort Pandas DataFrame? - GeeksforGeeks
Dec 2, 2024 · Pandas provides a powerful method called sort_values() that allows to sort the DataFrame based on one or more columns. The method can sort in both ascending and descending order, handle missing values, and even apply custom sorting logic.
Easy Steps to Sort Pandas DataFrames, Series, Arrays with …
4.2.1 Sorting a Pandas Series in an ascending order The following syntax enables us to sort the series in ascending order: >>> dataflair_se.sort_values(ascending=True)
NumPy Sorting Arrays - W3Schools
Sorting Arrays. Sorting means putting elements in an ordered sequence. Ordered sequence is any sequence that has an order corresponding to elements, like numeric or alphabetical, ascending or descending. The NumPy ndarray object has a …
pandas: Sort DataFrame/Series with sort_values(), sort_index()
Jan 25, 2024 · In pandas, the sort_values() and sort_index() methods allow you to sort DataFrame and Series. You can sort in ascending or descending order, or sort by multiple columns. Note that the older sort() method has been deprecated. Use reindex() to reorder rows and columns in any specific order. The pandas version used in this article is as follows.