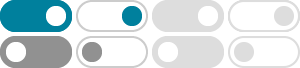
How do I sort strings that contain numbers in Java
Nov 17, 2016 · If you want to sort the strings as if they are numbers (i.e. "1" < "2" < "10"), then you need to convert the strings to numeric values. Depending on the range of these numbers, Integer.parseInt might do; you can always use BigInteger otherwise.
Java: Sort a numeric array and a string array - w3resource
Apr 1, 2025 · Write a Java program to sort an array of strings based on their length in ascending order. Write a Java program to sort an array of floating-point numbers using the selection sort algorithm. Write a Java program to sort an array of words by …
How to sort an Array of Strings in Java - GeeksforGeeks
Feb 16, 2024 · To sort an array of strings in Java, we can use Arrays.sort () function. We have an ArrayList to sort, we can use Collections.sort () In this method, the Arrays.stream (arrayOfStrings) creates a stream of strings from the array.
Arrays.sort() in Java - GeeksforGeeks
Apr 8, 2025 · To sort an array of strings in descending alphabetical order, the Arrays.sort() method combined with Collections.reverseOrder() method and it arranges the strings from Z to A based on lexicographical order.
Java: Sort a String array in a numeric way - Stack Overflow
May 9, 2013 · Write your own CustomStringComparator and use it with the sort method. @Override. public int compare(String str1, String str2) { // extract numeric portion out of the string and convert them to int. // and compare them, roughly something like this. int num1 = Integer.parseInt(str1.substring(0, str1.indexOf("%") - 1));
java - Sort a String array, whose strings represent int - Stack Overflow
Try a custom Comparator, like this: Arrays.sort(myarray, new Comparator<String>() { @Override. public int compare(String o1, String o2) { return Integer.valueOf(o1).compareTo(Integer.valueOf(o2)); });
7 Examples to Sort One and Two Dimensional String and Integer Array …
Aug 4, 2014 · You can use Arrays.sort () method to sort both primitive and object array in Java. But, before using this method, let's learn how the Arrays.sort () method works in Java. This will not only help you to get familiar with the API but also its inner workings.
Java Arrays. sort() Method - W3Schools
The sort() method sorts an array in ascending order. This method sorts arrays of strings alphabetically, and arrays of integers numerically.
How to Sort Strings Containing Numbers in Java?
Sorting strings that contain numbers in Java requires a custom comparator for correct numerical order. By default, sorting is done lexicographically, which may not yield the desired order when numeric values are present within the strings.
Sorting Arrays in Java - HowToDoInJava
Feb 4, 2022 · Learn to sort a Java array of primitives, strings and custom objects in multiple ways with the help of Comparable and Comparator interfaces, Arrays.sort() and Stream.sorted() APIs. We will learn to sort arrays in natural ordering , reverse ordering and any other custom ordering as …