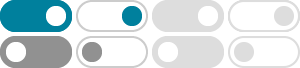
Program to sort an array of strings using Selection Sort
Feb 9, 2023 · Given an array of strings, sort the array using Selection Sort. Examples: Prerequisite : Selection Sort. // array of strings. Time Complexity: O (n 2), where n represents the size of the character array. Auxiliary Space: O (100), no extra space is …
Selection Sorting in Java with Strings? - Stack Overflow
Feb 27, 2014 · public static void selectionSortByName() throws IOException { String temp; for (int i = 0; i <= nameArraySize; i++){ String smallest = name[i]; for (int j = 0; j <= nameArraySize; j++){ if (name[j].compareTo(name[i]) < 0){ temp = smallest; name[j] = temp; name[i] = smallest; } } } }
Java Program for Selection Sort - GeeksforGeeks
Oct 23, 2024 · Selection Sort is a comparison-based sorting algorithm. It sorts an array by repeatedly selecting the smallest (or largest) element from the unsorted portion and swapping it with the first unsorted element.
Selection Sort (with Strings) - Central Connecticut State University
Here is selection sort with an array of String references: public static void selectionSort( String[] array ) { // Find the string reference that should go in each cell of. // the array, from cell 0 to the end. for ( int j=0; j < array.length-1; j++ ) { // Find min: the index of …
Selection Sorting String Arrays (Java) - Stack Overflow
Apr 21, 2011 · I need to know how to sort an array of Strings, I know how to do a selection sort, however I have BOTH numbers and letters in the array. The idea is to sort a hand of cards. This is what I have ...
Selection Sort - GeeksforGeeks
Dec 9, 2024 · Selection Sort is a comparison-based sorting algorithm. It sorts an array by repeatedly selecting the smallest (or largest) element from the unsorted portion and swapping it with the first unsorted element.
Selection Sort in Java - Tpoint Tech
Selection sort is an in-place sorting algorithm that sorts an array without the requirement of additional space, which is only a few variables for iteration. The space complexity of the selection sort is O(n), which implies that the space occupied by the algorithm does not vary depending on the size of the input array.
Selection Sort in String Program in Java - efaculty.in
Apr 4, 2022 · Selection Sort in String Program in Java. Write a program to input the names of 15 cities sort in descending order using selection sort technique.
write a program to sort string using Selection sort in java
In this core java programming tutorial we wrote a program to sort string using Selection sort in java. Example 1 to sort string using Selection sort in java> Input = java
java - Selection Sorting Algorithm for strings - Stack Overflow
Feb 12, 2015 · public void sortStrings(String[] strings) { for (int i = 0; i < strings.length; i++) swapMinFromIndex(strings, i); } This method starts by assuming the first element is the smallest. It then goes through the remaining elements and if any are smaller than the current smallest it stores their index.