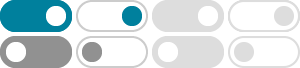
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · Given a string, the task is to print the given string in reverse order using recursion. Examples: Explanation: After reversing the input string we get “skeeG rof skeeG“. Explanation: After reversing the input string we get “noisruceR gnisU gnirts a esreveR“.
C++ Program To Print Reverse of a String Using Recursion
Jan 17, 2023 · Write a recursive function to print the reverse of a given string. Output: Explanation: Recursive function (reverse) takes string pointer (str) as input and calls itself with next location to passed pointer (str+1).
Write a recursive function that reverses the input string
Take the example "input" which should produce "tupni". You can reverse the string recursively by. If the string is empty or a single character, return it unchanged. Remove the first character. Reverse the remaining string. Add the first character above to the reversed string. Return the new string. @ArmenB.
Reversing A String in C++ (Using Recursion) - Stack Overflow
Mar 17, 2020 · Anyone can reverse a string one char at a time, but much cooler is to reverse each third of the string and swap the outer thirds. This cuts stack depth as well as sowing confusion amongst the competition. Note that max stack depth of recursion per character is N, whereas this is cube root of N.
Reverse a String – Complete Tutorial - GeeksforGeeks
Jan 29, 2025 · // C++ Program to reverse an array using Recursion #include <iostream> #include <vector> using namespace std; // recursive function to reverse a string from l to r void reverseStringRec (string & s, int l, int r) {// If the substring is empty, return if (l >= r) return; swap (s [l], s [r]); // Recur for the remaining string reverseStringRec (s ...
Reverse a string using recursion – C, C++, and Java
Apr 30, 2021 · Write a recursive program to efficiently reverse a given string in C, C++, and Java. For example, As seen in the previous post, we can easily reverse a given string using a stack data structure. As the stack is involved, we can easily convert the code to use the call stack. The implementation can be seen below in C and C++:
Reversing a string using with a recursive function in C++
Mar 29, 2013 · Recursivly reverses a string. @param return last_char, the last character currently in the string. @param go, the recursive function to return the character and continue within the function. **/ if (word.length()-1 > 0) char last_char = word[word.length()-1]; word.erase(word.length()-1); char go = string_reverse(word); return go; return false;
Different ways of reversing a string in C++ | Codecademy
Feb 5, 2025 · Learn multiple methods for reversing a string in C++, from basic manual approaches (like loops, recursion, stacks) to using STL (Standard Template Library) functions, and compare their performance.
C++ Recursion: Reversing a string using recursive function
Apr 14, 2025 · The "reverse_String()" function takes a reference to a string (str), the starting index (start), and the ending index (end) as parameters. Use recursion to reverse the string. The base case is when start >= end, indicating the string is fully reversed.
Reverse a String Recursively in C++ - Online Tutorials Library
Dec 23, 2019 · Learn how to reverse a string recursively in C++ with this comprehensive guide. Understand the recursive approach and see example code. Explore the process of reversing a string recursively in C++.