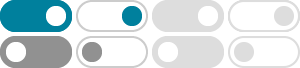
C# Recursion (With Examples) - Programiz
In C#, we can use recursion to find the factorial of a number. For example, class Program . public static void Main() . int fact, num; Console.Write("Enter a number: "); // take input from user . num = Convert.ToInt32(Console.ReadLine()); Program obj = new Program(); // calling recursive function . fact = obj.factorial(num);
Recursion in C# with Examples - Dot Net Tutorials
What is Recursion in C#? What does it mean by Recursive Function in C#? How does Recursion Work in C#? How do you trace a recursive function in C#? Example to Understand Recursion in C#; What are the advantages of Recursion in C# Language? What are the disadvantages of Recursion in C# Language?
Recursion in C# - GeeksforGeeks
Apr 26, 2025 · The process in which a function calls itself directly or indirectly is called recursion and the corresponding function is called a recursive function. A recursive algorithm takes one step toward solution and then recursively call itself to further move.
C# Recursive Functions | Useful Codes
Jan 1, 2025 · In this article, we explored the concept of C# Recursive Functions. We discussed the fundamental components of recursion, including the base case and recursive case, and presented common examples such as the Fibonacci sequence and binary search.
C# Back to Basics - Recursion and Recursive Methods - Code …
Aug 27, 2020 · Learn how to use Recursion and Recursive Methods in C#. And, what is the difference between regular methods and recursive ones.
C# Sharp programming exercises: Recursion - w3resource
Dec 20, 2024 · Write a program in C# Sharp to generate all possible permutations of an array using recursion. Test Data : Input the number of elements to store in the array [maximum 5 digits ] :3
Recursive Functions in C# - C# Corner
Answer: A recursive function is a function that calls itself. A function that calls another function is normal but when a function calls itself then that is a recursive function. Let's understand with an example how to calculate a factorial with and without recursion.
Recursive Methods in C#: A Guide for Beginners | C# Workshop
Here’s an example of a simple recursive method in C#: int factorial( int n) { if (n == 0 ) { return 1 ; // base case } else { return n * factorial(n - 1 ); // recursive call } }
Recursion in C# | Learn X By Example
This example demonstrates two types of recursive functions in C#: A static method Fact that calculates the factorial of a number. A local function Fib defined within the Main method that calculates Fibonacci numbers.
C# - Recursion Example - Dot Net Perls
Dec 8, 2023 · Recursive methods are used extensively in programming and in compilers. Recursion, notes. These algorithms help with complex problems. They solve problems and puzzles with brute force. They exhaust all possibilities. Example. Here is a recursive method. The method has 2 parameters, including a ref parameter.
- Some results have been removed