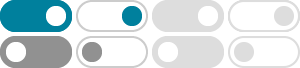
python - What is the complexity of the sorted () function? - Stack …
Feb 18, 2021 · Any comparison-based sort must take at least O(n log n). Without knowledge of the data and a specialized sort to take advantage of that (e.g. radix sort), this is the bound. It's not much of a stretch to say that a general (comparison-based) sort in a standard library will have this complexity bound. –
python - Time complexity in sort function - Stack Overflow
Oct 29, 2020 · i was solving a question in which expected time complexity was O(N) and i used .sort function. According to Timsort the time complexity is O(n logn). So if i used the .sort function ( as it uses Timsort function the time complexity is nlogn ) it should not have accepted my solution but it did accept –
Python sorting complexity on sorted list - Stack Overflow
May 22, 2014 · Python's sort is called timsort. Its average case performance is O(nlog(n)). Its average case performance is O(nlog(n)). It performs really well with pre-sorted lists because it's implementation is designed to seek out runs of sorted elements in the list.
Complexity of Python Sort Method - Stack Overflow
Sep 14, 2014 · If I have to sort some list, say a, using the sort method in Python such as below.. a=[3,7,1,0,2,8] a.sort() print a What are the worst, average and best cases of such programs in case of sorting ...
What algorithm does Python's built-in sort () method use?
Jan 30, 2023 · In early versions of Python, the sort function implemented a modified version of quicksort. However, in 2.3 this was replaced with an adaptive mergesort algorithm, in order to provide a stable sort by default.
What is the space complexity of the python sort?
Feb 13, 2018 · And even though according to the docs, the sort method sorts a list in place, it does use some additional space, as stated in the description of the implementation: timsort can require a temp array containing as many as N//2 pointers, which means as many as 2*N extra bytes on 32-bit boxes.
python - time complexity of sorting a dictionary - Stack Overflow
I was wondering what is the time complexity of sorting a dictionary by key and sorting a dictionary by value. for e.g : for key in sorted(my_dict, key = my_dict.get): <some-code> in the above line , what is the time complexity of sorted? If it is assumed that quicksort is used, is it O(NlogN) on an average and O(N*N) in the worst case ?
Time complexity of python sorted method inside a loop
Nov 3, 2017 · For each word in the list, I store the frequency of letters in a Counter as values which is then sorted using the method sorted(). I know that sorted() has a worst time complexity of O(nlog(n)). Am I right to say that the worst time complexity of the whole code is O(n^2 log(n)) since sorted() is used inside a for loop?
python - Time complexity in sorting a list by converting it to a set ...
Apr 8, 2020 · Now: according to the time-complexity section of python.org and in more detail here it is stated that the average time-complexity of adding an element to a set is O(1). This means if you have an unsorted list of integers it should be possible to sort them by simply doing:
sorting - How does Python sort() time complexity change with …
May 21, 2021 · I need to sort the array in the lexicographical order of the string contents (excluding the serial number). If two strings in the array have the same content, I need to sort them based on their serial numbers. I used the sort() function in combination with a lambda function which in turn uses the split() function.