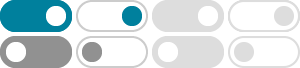
Practice Python
Welcome to Practice Python! There are over 40 beginner Python exercises just waiting to be solved. Each exercise comes with a small discussion of a topic and a corresponding post with a solution. Follow on Feedly, Twitter, our mailing list, or your favorite RSS reader.
Exercises and Solutions - Practice Python
Beginner Python exercises. Home; Why Practice Python? Why Chilis? Resources for learners; All Exercises. 1: Character Input 2: Odd Or Even 3: List Less Than Ten 4: Divisors 5: List Overlap 6: String Lists 7: List Comprehensions 8: Rock Paper Scissors 9: Guessing Game One 10: List Overlap Comprehensions 11: Check Primality Functions 12: List Ends 13: Fibonacci 14: List Remove Duplicates
40 Error Checking - Practice Python
Jul 17, 2022 · It also makes the code clear to understand which error we CAN handle and which we CANNOT. In Python, when the program throws an error, that error is called an Exception . The mechanism to “catch” an error in the program is called a try / catch block.
01 Character Input - Practice Python
Jan 29, 2014 · User input in Python. To get user input in Python (3), the command you use is input(). Store the result in a variable, and use it to your heart’s content. Remember that the result you get from the user will be a string, even if they enter a number. For example, name = input ("Give me your name: ") print ("Your name is "+ name)
21 Write To A File - Practice Python
Nov 30, 2014 · Python makes it very easy to write to a file. Depending on what kind of file you want to write to and what kind of data you are writing, your options are plenty. I will show you the simplest form of writing to a file - writing plain text to a plain old text file.
f Strings - Practice Python
Mar 6, 2022 · Instead, there is a built-in Python functionality called f-strings, short for formatted string literals that solve the problem for us! Variables For me, the most common use of f-strings is to automatically display variables in a string.
07 List Comprehensions - Practice Python
Mar 19, 2014 · Write one line of Python that takes this list a and makes a new list that has only the even elements of this list in it. Discussion. Concepts for this week: List comprehensions; List comprehensions. The idea of a list comprehension is to make code more compact to accomplish tasks involving lists. Take for example this code:
02 Odd Or Even - Practice Python
Feb 5, 2014 · A fundamental thing you want to do with your program is check whether some number is equal to another. Say the user tells you how many questions they answered incorrectly on a practice exam, and depending on the number of correctly-answered questions, you can suggest a specific course of action.
08 Rock Paper Scissors - Practice Python
Mar 26, 2014 · The output of this code segment is: 5 4 3 2 1. A particularly useful way to use while loops is checking user input for correctness. For example: quit = input ('Type "enter" to quit:') while quit!= "enter": quit = input ('Type "enter" to quit:') The uses for this are infinite, and can (and should!) be combined with conditionals to yield the most ...
29 Tic Tac Toe Game - Practice Python
Aug 3, 2016 · In 3 previous exercises, we built up a few components needed to build a Tic Tac Toe game in Python: Draw the Tic Tac Toe game board; Checking whether a game board has a winner; Handle a player move from user input; The next step is to put all these three components together to make a two-player Tic Tac Toe game!