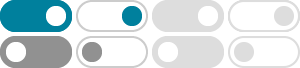
Searching Algorithms for 2D Arrays (Matrix) - GeeksforGeeks
Apr 14, 2025 · Linear Search is the simplest technique for finding an element. In the case of an unsorted 2D matrix, we must traverse each cell one by one to check for the target element.
How to search for an element in a 2d array in Python
Jun 5, 2021 · def searchTDArray(array, value): pos = [] for i in range(len(array)): # Return number of rows. for j in range(len(array[0])): # Return number of columns.
Linear Search – Python | GeeksforGeeks
Apr 7, 2025 · The article explains how to find the index of the first occurrence of an element in an array using both iterative and recursive linear search methods, returning -1 if the element is not found.
Search in two dimensional array in Python - Stack Overflow
I'd like to be able to retrieve specifics rows in a large dataset (9M lines, 1.4 GB) given two or more parameters through Python. For example, from this dataset : ID1 2 10 2 2 1 2 2 ...
Search in 2D list using python to find x,y position
Aug 4, 2013 · If your "2D" list is rectangular (same number of columns for each line), you should convert it to a numpy.ndarray and use numpy functionalities to do the search. For an array of integers, you can use == for comparison. For an array of float numbers, you should use np.isclose instead: a = np.array(c, dtype=int) i,j = np.where(a == element) or
Python | Using 2D arrays/lists the right way - GeeksforGeeks
Jun 20, 2024 · Using 2D arrays/lists the right way involves understanding the structure, accessing elements, and efficiently manipulating data in a two-dimensional grid. When working with structured data or grids, 2D arrays or lists can be useful. A 2D array is essentially a list of lists, which represents a table-like structure with rows and columns.
Search in a Row-wise and Column-wise Sorted 2D Array in Python …
Learn how to search in a row-wise and column-wise sorted 2D array in Python. We'll implement divide and rule to reduce time complexity.
Python Program For Linear Search (With Code & Explanation) - Python …
Linear search is a simple searching algorithm that traverses through a list or array in a sequential manner to find a specific element. In linear search, we compare each element of the list with the target element until we found a match or we have traversed the entire list.
Implement linear search using one and two dimensional array
Linear Search with One-Dimensional Array. To implement linear search with a one-dimensional array, you can iterate through each element in the array and compare it with the target value until a match is found or the end of the array is reached. Here's a simple example in Python:
Search a 2D Matrix - LeetCode
Search a 2D Matrix. You are given an m x n integer matrix matrix with the following two properties: Each row is sorted in non-decreasing order. The first integer of each row is greater than the last integer of the previous row. Given an integer target, return true …