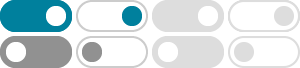
Implementation of Stack in JavaScript - GeeksforGeeks
Feb 11, 2025 · What is a Stack? A stack is a linear data structure that allows operations to be performed at one end, called the top. The two primary operations are: Push: Adds an element to the top of the stack. Pop: Removes and returns the top element from the stack. Occurs when you try to perform a pop or peek operation on an empty stack.
How do you implement a Stack and a Queue in JavaScript?
An array is a stack in Javascript. Just use arr.push(x) and y = arr.pop(). Here is the simplest method for implementing a queue in Javascript that has an amortized time of O(1) for both enqueue(x) and y = dequeue(). It uses a mapping from insertion index to element.
Implementing Javascript Stack Using an Array - JavaScript Tutorial
In this tutorial, you will learn how to implement the JavaScript stack data structure using the array push and pop methods.
JavaScript Program to Implement a Stack
In this example, you will learn to write a JavaScript program that will implement a stack.
How to implement a stack in vanilla JavaScript and ES6
Dec 2, 2018 · We are going to implement a stack with a JavaScript array which has inbuilt methods like push and pop. Making the properties and methods private with closure and IIFE (Immediately Invoked Function Expression). Stack using ES6. Stack using ES6 WeakMap.
Stacks in JavaScript: A Simple Guide | by devtalib | Medium
Jan 22, 2024 · In JavaScript, a stack is usually made using an array. Let’s look at a simple example: Imagine you’re making a stack for numbers. Here’s how you can do it: return "Oops, the stack is...
The Stack — what is it exactly, and how does it work in JavaScript?
May 3, 2020 · Today we’re going to be diving deeper into the stack to gain a better understanding of how the JavaScript engine processes blocks of code when a script is executed. We’ll be taking a closer...
Understanding Stack Data Structure: A Step-by-Step Guide to ...
Oct 8, 2024 · What is a Stack? A Stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of it like a stack of books: you can only add or remove books from the top of the stack. Pros and Cons of Using Stack
Stacks in JavaScript. Don’t use an Array when a Stack will do | by ...
Aug 13, 2020 · Even though there is no built-in stack data structure in JavaScript, we use array data structure with the help of the push(), pop(), unshift(), and shift() methods to create stacks. Amongst...
Implement a stack in JavaScript - Techie Delight
Oct 8, 2023 · This post will discuss how to implement stack data structure in JavaScript. A stack is a data structure that follows the principle of Last In First Out (LIFO), meaning that the last element that is added to the stack is the first one that is removed.
- Some results have been removed