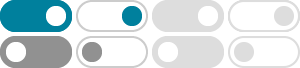
How to convert a string to an integer in JavaScript?
If your string is already in the form of an integer: var x = +"1000"; floor() If your string is or might be a float and you want an integer: var x = Math.floor("1000.01"); // floor() automatically converts string to number Or, if you're going to be using Math.floor several times: var floor = Math.floor; var x = floor("1000.01"); parseFloat()
JavaScript Type Conversions - W3Schools
JavaScript variables can be converted to a new variable and another data type: The global method Number() converts a variable (or a value) into a number. A numeric string (like "3.14") converts to a number (like 3.14). An empty string (like "") converts to 0. A non numeric string (like "John") converts to NaN (Not a Number). These will convert:
JavaScript parseInt() Method - W3Schools
The parseInt method parses a value as a string and returns the first integer. A radix parameter specifies the number system to use: 2 = binary, 8 = octal, 10 = decimal, 16 = hexadecimal. If radix is omitted, JavaScript assumes radix 10. If the value begins with "0x", JavaScript assumes radix 16.
parseInt() - JavaScript | MDN - MDN Web Docs
Apr 3, 2025 · For example, parseInt("42") and parseFloat("42") would return the same value: a Number 42. The parseInt function converts its first argument to a string, parses that string, then returns an integer or NaN. If not NaN, the return value will be the integer that is the first argument taken as a number in the specified radix.
Fastest way to cast a float to an int in javascript?
Dec 3, 2015 · In javascript, what function floatToInt(x) has the fastest running time such that floatToInt(12.345) returns 12? Are there multiple functions that do your job? Just use Math.round (or Math.floor, depending what you need). Is the speed of your conversion really worth making less readable code?
Convert a String to an Integer in JavaScript | GeeksforGeeks
Nov 12, 2024 · These are the following ways to convert string to an integer in JavaScript: 1. Using Number () Method. The number () method converts a string into an integer number. It works similarly to the unary plus operator. 2. Using Unary + Operator. The Unary + Operator is a quick and concise way to convert strings to numbers.
How to Convert a String to a Number in JavaScript
May 2, 2022 · In this article, I will show you 11 ways to convert a string into a number. One way to convert a string into a number would be to use the Number() function. In this example, we have a string called quantity with the value of "12". If we used the typeof operator on quantity, then it will return the type of string.
7 ways to convert a String to Number in JavaScript
Dec 16, 2020 · If you need an integer, use parseInt. If you need a floating-point number, use parseFloat. Number() is a good general-purpose option, while the unary plus operator and multiplication by 1 are convenient for quick conversions but might have unexpected behavior.
JavaScript Convert String to Number – JS String to Int Example
Jun 29, 2022 · Both the parseInt() and parseFloat() functions takes in a string as a parameter, and then convert that string to an integer/number. You can also use parseInt() for converting a non-integer number to an integer, while parseFloat() is the more powerful method as it can maintain floats and some mathematical logic:
String to Number in JavaScript – Convert a String to an Int in JS
Sep 5, 2024 · JavaScript has different built-in methods to convert or cast a string into a number. In this article, you will learn some of the built-in JavaScript methods available for converting strings to numbers, along with an introduction (or refresher!) to the basics of how strings and numbers work in JavaScript.