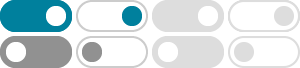
Take user input into vector in C++ - CodeSpeedy
The basic way is if the user will give the size of the vector then we can take input into vector simply using for loop. See the code below to understand it better. Input: 5 2 9 4 7 2
Iterate through a C++ Vector using a 'for' loop - Stack Overflow
Oct 3, 2012 · Iterating vector using auto and for loop. vector<int> vec = {1,2,3,4,5} for(auto itr : vec) cout << itr << " "; Output: 1 2 3 4 5 You can also use this method to iterate sets and list. Using auto automatically detects the data type used in the template and lets you use it.
How to store user input (cin) into a Vector? - Stack Overflow
You probably want to read in more numbers, not only one. For this, you need a loop. int main() { int input = 0; while(input != -1){ vector<int> V; cout << "Enter your numbers to be evaluated: " << endl; cin >> input; V.push_back(input); write_vector(V); } return 0; }
taking input into vector using for-range loop - Stack Overflow
Jun 23, 2021 · std::vector<int> vec(c); for(auto& i : ivec){ cin>>i; } This creates a vector with size c and modifies its element. Or. std::vector<int> vec; for(int i=0; i<c; ++i) { int temp; std::cin >> temp; vec.push_back(temp); } where this creates an empty vector and pushes the element at the end. See cppreference for more info.
How to Iterate Through a Vector in C++? - GeeksforGeeks
Nov 15, 2024 · Iterating or traversing a vector means accessing each element of the vector sequentially. In this article, we will learn different methods to iterate over a vector in C++. The simplest way to iterate a vector is by using a range based for loop .
How to Use a Range-Based for Loop with a Vector in C++?
Feb 5, 2024 · In this article, we'll look at how to iterate over a vector using a range-based loop in C++. Example. Input: myVector: {1, 2, 3, 4, 5} Output: 1 2 3 4 5 Range-Based for Loop with Vector in C++. The syntax of range-based for loop is: for (const auto &element : container_name) {// Code to be executed for each element} We can use this syntax with ...
How to take take user input into vector in CPP - CodersPacket
Jul 31, 2024 · Use a for loop to iterate n times. Within each iteration, read an element from the user and store it in the element variable. Add the element to the vect1 using the push_back() function.
How to Iterate Through a Vector in C++ - Delft Stack
Feb 12, 2024 · We can use the for loop to iterate through a vector in C++. Syntax: statements. We first declare an index with a value of 0. The loop will run till the time the index is less than the size of the vector, and we will increment it with a value of …
How to Traverse a Vector using for_each Loop in C++?
Mar 18, 2024 · In this article, we will learn how we can use a for_each loop to traverse elements of a vector in C++. Example Input: myVector = {1,2,3,4,5} Output: // Squared each element of vector and traversal. myVector ={1,4,9,16,25}
Getting user input in a for loop - C++ Forum - C++ Users
Sep 19, 2017 · Use an array, or better, a vector. int main() int N; cout << "Please Enter N: "; cin >> N; vector<int> store(N); for (int i = 0; i < N; i++) cout << "Integer #" << i+1 << " "; cin >> store[i]; cout << "\n\nHere are the numbers:\n"; for (size_t i=0; …