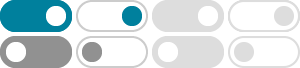
How do you initialize an array in C#? - Stack Overflow
Since C# 12, we can use collection expressions: // Create an array: int[] a = [1, 2, 3, 4, 5, 6, 7, 8]; // Create a jagged 2D array: int[][] twoD = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]; // Create a jagged 2D array from variables: int[] row0 = [1, 2, 3]; int[] row1 = [4, 5, 6]; int[] row2 = [7, 8, 9]; int[][] twoDFromVariables = [row0, row1, row2];
c# - All possible array initialization syntaxes - Stack Overflow
Feb 10, 2018 · What are all the array initialization syntaxes that are possible with C#? These are the current declaration and initialization methods for a simple array. Note that other techniques of obtaining arrays exist, such as the Linq ToArray() extensions on IEnumerable<T>.
The array reference type - C# reference | Microsoft Learn
Dec 14, 2024 · Many of the examples in this article use collection expressions (which use square brackets) to initialize the arrays. Collection expressions were first introduced in C# 12, which shipped with .NET 8. If you can't upgrade to C# 12 yet, use {and } to initialize the arrays instead.
Different Ways to Initialize Arrays in C# - Code Maze
Jul 13, 2022 · In this article, we have learned how to initialize arrays in C# using different techniques. Additionally, we’ve shown several tests to prove the size of our initialized arrays.
How to populate/instantiate a C# array with a single value?
For large arrays or arrays that will be variable sized you should probably use: Enumerable.Repeat(true, 1000000).ToArray(); For small array you can use the collection initialization syntax in C# 3: bool[] vals = new bool[]{ false, false, false, false, false, false, false };
C# Arrays - W3Schools
In C#, there are different ways to create an array: It is up to you which option you choose. In our tutorial, we will often use the last option, as it is faster and easier to read. However, you should note that if you declare an array and initialize it later, …
C# Array: How To Declare, Initialize And Access An Array In C#?
Apr 1, 2025 · Learn All About C# Array in This In-depth Tutorial. It Explains How To Declare, Initialize And Access Arrays Along with Types And Examples Of Arrays in C#.
C# Arrays (With Examples) - Programiz
We can use the index number to initialize an array in C#. For example, // declare an array int[] age = new int[5]; //initializing array age[0] = 12; age[1] = 4; age[2] = 5; ...
Array Initialization in C#: Detailed Guide (2025) - ByteHide
Dec 21, 2023 · Exploring How to Initialize an Array in C#. The process of initializing an array in C# is a fundamental programming concept, yet it encompasses a variety of methods with different levels of complexity.
Arrays in C#: Create, Declare and Initialize the Arrays - ScholarHat
Jan 26, 2025 · You can use shorthand initialization to create and initialize an array in one line. For example, you can declare and initialize an array of integers like this: int [] numbers = { 1 , 2 , 3 , 4 , 5 };