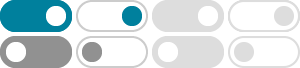
c++ - Reverse Contents in Array - Stack Overflow
reverse(arr, SIZE); return 0; int temp; for (int i = 0; i < count/2; ++i) arr[i] = temp; temp = arr[count-i-1]; arr[count-i-1] = arr[i]; arr[i] = temp; cout << temp << " "; Your loop only runs for half the length of the array when you divide the count by 2, so it only prints half of the array contents to the screen. Save this answer.
Array Reverse – Complete Tutorial - GeeksforGeeks
Sep 25, 2024 · Given an array arr[], the task is to reverse the array. Reversing an array means rearranging the elements such that the first element becomes the last, the second element becomes second last and so on. Examples: Input: arr[] = {1, 4, 3, 2, 6, 5} Output: {5, 6, 2, 3, 4, 1}Explanation: The first eleme
c++ - how to print an array backwards - Stack Overflow
Jun 15, 2015 · #include<iostream> using namespace std; int main () { int a[10], x, i; cout << "enter the size of array" << endl; cin >> x; cout << "enter the element of array" << endl; for (i = 0; i < x; i++) { cin >> a[i]; } cout << "reverse of array" << endl; for (i = x - 1; i >= 0; i--) cout << a[i] << endl; }
How to Reverse an Array using STL in C++? - GeeksforGeeks
Nov 15, 2024 · In this article, we will learn how to reverse an array using STL in C++. The most efficient way to reverse an array using STL is by using reverse () function. Let’s take a look at a simple example: This method reverses the array in-place i.e. it modifies the original array and rearranges it in reverse order.
Reverse an Array in C++ [3 Methods] - Pencil Programmer
There are multiple ways to reverse an array in C++. Let’s discuss each of them. The idea is to create a new array and assign elements to it from the input array in reversed order. To do this, we simply have to: Initialize an array with values. Declare a new empty array of the same size.
C++ Program to Reverse an Array - CodesCracker
This article will teach you how to reverse an array entered by the user during runtime in a C++ program. Here is the list of programs for reversing an array: Print an array's inverse without actually reversing it; Reverse an array, and then print; Reverse an array using the pointer; Reverse an array using a user-defined function
Reverse Array Elements In-Place in C++ - Online Tutorials Library
Oct 7, 2021 · Learn how to reverse the elements of an array in-place using C++. This guide provides a step-by-step approach with code examples.
Program to reverse an array using pointers - GeeksforGeeks
Jan 13, 2023 · Given an array arr[] and an integer k, the task is to reverse every subarray formed by consecutive K elements. Examples: Input: arr[] = [1, 2, 3, 4, 5, 6, 7, 8, 9], k = 3 Output: 3, 2, 1, 6, 5, 4, 9, 8, 7 Input: arr[] = [1, 2, 3, 4, 5, 6, 7, 8], k = 5 Output: 5, 4, 3, 2, 1, 8, 7, 6 Input: arr[] = [1
Reverse an Array in C++ - Tpoint Tech - Java
Mar 17, 2025 · Following are the various ways to get the reverse array in the C++ programming language. Reverse an array using for loop; Reverse an array using the reverse() function; Reverse an array using the user-defined function; Reverse an array using the pointers; Reverse an array using the Recursion function; Program to reverse an array using the for ...
How to Reverse Array in C++ - Delft Stack
Mar 12, 2025 · This article demonstrates how to reverse an array in C++. Explore various methods including loops, the STL's `std::reverse`, and recursion. Learn through clear examples and detailed explanations to enhance your C++ programming skills.