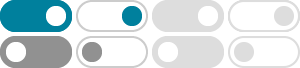
java - Take a char input from the Scanner - Stack Overflow
Dec 19, 2012 · There is no API method to get a character from the Scanner. You should get the String using scanner.next() and invoke String.charAt(0) method on the returned String. Scanner reader = new Scanner(System.in); char c = reader.next().charAt(0); Just to be safe with whitespaces you could also first call trim() on the string to remove any whitespaces.
Scanner and nextChar() in Java - GeeksforGeeks
Jan 4, 2025 · In Java, the Scanner class is present in the java.util package is used to obtain input for primitive types like int, double, etc., and strings. We can use this class to read input from a user or a file. In this article, we cover how to take different …
How can I enter "char" using Scanner in java? - Stack Overflow
Apr 16, 2014 · You could take the first character from Scanner.next: char c = reader.next().charAt(0); To consume exactly one character you could use: char c = reader.findInLine(".").charAt(0); To consume strictly one character you could use: char c = reader.next(".").charAt(0);
Reading a single char in Java - Stack Overflow
Aug 29, 2010 · You can use Scanner like so: Scanner s= new Scanner(System.in); char x = s.next().charAt(0); By using the charAt function you are able to get the value of the first char without using external casting.
Java Scanner Taking a Character Input - Baeldung
Jan 8, 2024 · Let’s see how we can use the next() method of Scanner and the charAt() method of the String class to take a character as input: @Test public void givenInputSource_whenScanCharUsingNext_thenOneCharIsRead() { Scanner sc = new Scanner(input); char c = sc.next().charAt(0); assertEquals('a', c); }
How to Read Character in Java - Tpoint Tech
Apr 26, 2025 · To read a character in Java, we use next () of the Scanner class method followed by chatAt () at method of the String class. The next () method is a method of Java Scanner class. It finds and returns the next complete token from this scanner. A complete token is preceded and followed by input that matches the delimiter pattern.
Java User Input (Scanner class) - W3Schools
Java User Input. The Scanner class is used to get user input, and it is found in the java.util package. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read Strings:
Using Java Scanner for Character Input: A Comprehensive Guide
This tutorial provides a detailed guide on using the Java Scanner class to read character input. It covers everything from basic character input to advanced techniques for handling user input effectively.
How to Get a Char From the Input in Java - Delft Stack
Mar 11, 2025 · In this article, we will explore various methods to read a character from input in Java. We’ll cover everything from using the Scanner class to employing BufferedReader, ensuring you have a comprehensive understanding of each approach.
Java Scanner (With Examples) - Programiz
The Scanner class of the java.util package is used to read input data from different sources like input streams, users, files, etc. In this tutorial, we will learn about the Java Scanner and its methods with the help of examples.