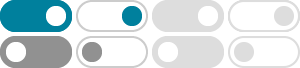
C# Creating an array of arrays - Stack Overflow
I think you may be looking for Jagged Arrays, which are different from multi-dimensional arrays (as you are using in your example) in C#. Converting the arrays in your declarations to jagged arrays should make it work. However, you'll still need to use two loops to iterate over all the items in the 2D jagged array.
How do you initialize an array in C#? - Stack Overflow
Aug 6, 2009 · Read this: Arrays (C# Programming Guide) //can be any length int[] example1 = new int[]{ 1, 2, 3 }; //must have length of two int[] example2 = new int[2]{1, 2 ...
How to declare an array of objects in C# - Stack Overflow
Default for reference types is null => you have an array of nulls. You need to initialize each member of the array separatedly. houses[0] = new GameObject(..); Only then can you access the object without compilation errors. So you can explicitly initalize the array: for (int i = 0; i < houses.Length; i++) { houses[i] = new GameObject(); }
Adding values to a C# array - Stack Overflow
Aug 24, 2018 · The method will make adding 400 items to the array create a copy of the array with one more space and moving all elements to the new array, 400 hundred times. so is not recommended performance wise. – KinSlayerUY
Initializing a C# array with multiple copies of the same element
I want to do a similar thing in C#, more specifically creating an array of n doubles with all elements initialized to the same value x. I have come up with the following one-liner, relying on generic collections and LINQ: double[] v = new double[n].Select(item => x).ToArray();
How do I initialize an empty array in C#? - Stack Overflow
Jan 4, 2012 · When you declare an array of specific size, you specify the fixed number of slots available in a collection that can hold things, and accordingly memory is allocated. To add something to it, you will need to anyway reinitialize the existing array (even if you're resizing the array, see this thread). One of the rare cases where you would want to ...
c# - All possible array initialization syntaxes - Stack Overflow
Feb 10, 2018 · The array creation syntaxes in C# that are expressions are: new int[3] new int[3] { 10, 20, 30 } new int[] { 10, 20, 30 } new[] { 10, 20, 30 } In the first one, the size may be any non-negative integral value and the array elements are initialized to the default values.
Declaring and Using Global Arrays c# - Stack Overflow
I couldn't find any information pertaining to this question. I am trying to create a global array in C# so that I can input information into it at different points in my code and then call the information back at a later time to display it. For example, I want to …
How to Fill an array from user input C#? - Stack Overflow
Oct 23, 2008 · after showing the user a msg to input the elements(6 floating numbers) I want the user to input the elements separeated by commas so at first i decleared the array double[] array; array = new double[6]; now i need to read the elements –
c# - Options for initializing a string array - Stack Overflow
Sep 3, 2013 · Seperate string insert to array c#-6. need to convert code for pick random line and display. Related. 12183.