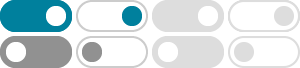
Retrieving the text of the selected <option> in <select> element
Mar 4, 2009 · How can I get the text of the selected option (i.e. "Test One" or "Test Two") using JavaScript. document.getElementsById('test').selectedValue returns 1 or 2, what property returns the text of the selected option? var elt = document.getElementById(elementId); if (elt.selectedIndex == -1) return null; return elt.options[elt.selectedIndex].text;
html - Get selected option text with JavaScript - Stack Overflow
There are two solutions, as far as I know. // log(`e.target`, e.target); const select = e.target; const value = select.value; const desc = select.selectedOptions[0].text; log(`option desc`, desc); <label for="area">Area</label> <select id="area"> <option value="101">A1</option> <option value="102">B2</option> <option value="103">C3</option>
Get selected value/text from Select on change - Stack Overflow
Nov 1, 2017 · If you wanted the text (e.g. -Select- or Communication) you would use text: select.text or in jQuery select.text (). I would not rely on getElementById as you can potentially use the same method for different selects. I'd look at the answer proposed by YakovL if that is what you are looking for.
How to get the Highlighted/Selected text in JavaScript?
Apr 19, 2023 · Method 1: window.getSelection property: The window.getSelection method returns a selection object that represents the text selection made by the user or the caret’s current position. Syntax: return window.getSelection();
How to Get Selected Value in Dropdown List using JavaScript?
Oct 8, 2024 · To get the selected value from dropdown list, we need to use JavaScript. The HTML DOM value property is used to get the selected value of a dropdown list item. Syntax. Example: The below code uses the value property to get the value of the selected dropdown item. Get Selected Value in Dropdown. List using JavaScript.
HTML <select> Tag - W3Schools
Create a drop-down list with four options: More "Try it Yourself" examples below. The <select> element is used to create a drop-down list. The <select> element is most often used in a form, to collect user input. name attribute, no data from the drop-down list will be submitted).
How to Get the Value of Selected Option in a Select Box
To get the value of the selected option in a select box using JavaScript, you can use the value property of the select element. Here's an example: var selectedValue = selectBox.value; alert(selectedValue); // prints the value of the selected option to …
Get selected text from a drop-down list with JavaScript/jQuery
Apr 24, 2024 · This post will discuss how to get selected text from a dropdown list in JavaScript and jQuery. 1. Using jQuery. With jQuery, you can use the text() or html() method to get the selected text from a dropdown. This can be done in several ways using the :selected property to get the chosen option of the select element, as shown below:
How to Retrieve the Text of the Selected option Element in a select ...
Mar 25, 2022 · In this article, we’ll look at how to retrieve the text of the selected option element in a select element with JavaScript. We can get the index of the selected item with the selectedIndex property. Then we can use the text property to get the text of the selected item. For instance, if we have the following HTML: <option value="1">apple</option>
Get the Value/Text of Select or Dropdown on Change using JS
Mar 5, 2024 · To get the value and text of a select element on change: Add a change event listener to the select element. Use the value property to get the value of the element, e.g. select.value. Use the text property to get the text of the element, e.g. select.options[select.selectedIndex].text. Here is the HTML for the examples.