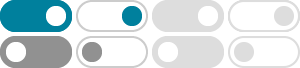
Check if something is (not) in a list in Python - Stack Overflow
How do I check if something is (not) in a list in Python? The cheapest and most readable solution is using the in operator (or in your specific case, not in). As mentioned in the documentation, The operators in and not in test for membership. x in s evaluates to True if x is a member of s, and False otherwise. x not in s returns the negation of ...
python - Check if item is in an array / list - Stack Overflow
If I've got an array of strings, can I check to see if a string is in the array without doing a for loop? Specifically, I'm looking for a way to do it within an if statement, so something like this: if [check that item is in array]:
Check if string does not contain strings from the list
Oct 31, 2019 · To ensure the input string does not contain any char from the list, your condition should be:... if not any(x in mystr for x in mylist):
Find element in Array – Python | GeeksforGeeks
Nov 28, 2024 · in operator is one of the most straightforward ways to check if an item exists in an array. It returns True if the item is present and False if it's not. Let's take a look at methods of finding an item in an array: If we want to find the index of an item in the array, we can use the index () method.
Python: Check if Array/List Contains Element/Value - Stack Abuse
Feb 27, 2023 · In this tutorial, we've gone over several ways to check if an element is present in a list or not. We've used the for loop, in and not in operators, as well as the filter() , any() and count() methods.
Check if element exists in list in Python - GeeksforGeeks
Nov 29, 2024 · In this article, we will explore various methods to check if element exists in list in Python. The simplest way to check for the presence of an element in a list is using the in Keyword. Example: [GFGTABS] Python a = [10, 20, 30, 40, 50] # Check if 30 exists in the list if 30 in a: print("Eleme
Python – Test if string contains element from list - GeeksforGeeks
Jan 8, 2025 · Testing if string contains an element from list is checking whether any of the individual items in a list appear within a given string. Using any() with a generator expression any() is the most efficient way to check if any element from the list is present in the list.
Python: check if item or value exists in list or array
Apr 1, 2019 · How to check if element exists in array (or list) using Python and the operator in, or calling the method index; handling the exception
How to Check if an Array Contains a Value in Python? - Python …
Dec 26, 2024 · Learn how to check if an array (or list) contains a value in Python. Explore methods like in, loops, and NumPy for efficient, step-by-step solutions with examples
Check if something is (not) in a list in Python - W3docs
Similarly, you can check if an item is not in a list using the not in keyword. For example: if 5 not in my_list: print ("5 is not in the list") else: print ("5 is in the list") This will print "5 is not in the list" because the number 5 is not in the list.