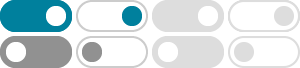
java - Creating an instance using the class name and calling ...
Class<?> clazz = Class.forName(className); Constructor<?> ctor = clazz.getConstructor(String.class); Object object = ctor.newInstance(new Object[] { ctorArgument }); That will only work for a single string parameter of course, but you can modify it pretty easily. Note that the class name has to be a fully-qualified one, i.e. including the ...
How to create a custom exception type in Java? - Stack Overflow
(1) You should make your new exception a public one, so that methods calling your method may catch this new exception and do something with it (if you mean this new exception to be a checked exception, see my edit. (2) Make the constructors public, no need to hide them. (3) You can get rid of the constructors you don't need, all 4 are not ...
What are all the different ways to create an object in Java?
Sep 18, 2008 · This is the most common way to create an object in java. Almost 99% of objects are created in this way. Employee object = new Employee(); Method 2. Using Class.forName(). Class.forName() gives you the class object, which is useful for reflection. The methods that this object has are defined by Java, not by the programmer writing the class.
java - Creating new classes from code - Stack Overflow
Jun 7, 2011 · Either use BCEL to create byte code and class files (the hard way) or create the source code in memory and use the Java 6 Compiler API (that's what I would do). But with Compiler API you need a Java SDK while running the application, a JRE is not sufficient. Further Reading. The Java 6.0 Compiler API (There a lot of tutorials on the web)
java - "What are the criteria for creating a new class? Should there …
May 11, 2014 · Usually one extends an existing class when the new one adds either new state or behavior. Toy feels very generic to me. Could it be abstract, with Ball providing its special implementation of an abstract method? If not, you sound like a …
java - IntelliJ how do I generate a new class? - Stack Overflow
IntelliJ will offer the option to create new class in the new menu, only if you right click within the part of your project structure, which is marked as Sources root or Test sources root. In your case, right click the src/main directory in the project structure view and select Mark directory As/Sources Root. That should fix it.
How do I create a new class in IntelliJ without using the mouse?
Feb 12, 2010 · If you are already in the Project View, press Alt+Insert (New) | Class. Project View can be activated via Alt+1. To create a new class in the same directory as the current one use Ctrl+Alt+Insert (New...). You can also do it from the Navigation Bar, press Alt+Home, then choose package with arrow keys, then press Alt+Insert.
java - Why some classes don't need the word "New" when …
Sep 4, 2012 · The only way to create a new object in Java is with new [1]. However, in some classes, you're not permitted to say new for yourself, you must call a factory method, which might be static (as with your logger example) or not. The author of a class sets this up by making the constructor(s) have access other than public.
How would I create a new object from a class using a for loop in …
Oct 1, 2013 · I have a class named Card and I have this for loop: int i; for (i = 0; i < 13; i++) { Card cardNameHere = new Card(); } What I want to do is create new instances based on the for loop. So for example, I would like the names to be card1, card2, card3, etc. The number would come from the for loop. I have tried this and it does not seem to work:
Java how to instantiate a class from string - Stack Overflow
Jan 21, 2012 · If I want to instantiate a class that I retrieved with forName(), I have to first ask it for a java.lang.reflect.Constructor object representing the constructor I want, and then ask that Constructor to make a new object. The method getConstructor(Class[] parameterTypes) in Class will retrieve a Constructor; I can then use that Constructor by ...