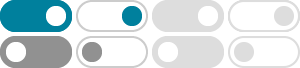
Prim's Algorithm with a Java Implementation - GeeksforGeeks
Jun 13, 2024 · In this article we explain about How to implement Prim's Algorithm with Java programming with required operations like adding edges, Graph Initialization and Setting the Starting Point in Prim's Algorithm, Building the MST and other operations.
Prim’s Algorithm for Minimum Spanning Tree (MST)
Feb 26, 2025 · Follow the given steps to utilize the Prim’s Algorithm mentioned above for finding MST of a graph: Create a set mstSet that keeps track of vertices already included in MST. Assign a key value to all vertices in the input graph.
Prim's MST algorithm implementation with Java - Stack Overflow
Edge class can create a new Edge that has gets parameters (Vertex start, Vertex end, int edgeWeight). The class has methods to return the usual info like start vertex, end vertex and the weight. Graph class reads data from a text file and adds new Edges to an ArrayList.
Prim’s Algorithm with a Java Implementation - Baeldung
Jan 25, 2024 · Prim’s algorithm takes a weighted, undirected, connected graph as input and returns an MST of that graph as output. It works in a greedy manner . In the first step, it selects an arbitrary vertex.
sangamsahai/Minimum-Spanning-Tree--Prims-Algorithm---Java ... - GitHub
This is a Java implementation of Prims Algorithm for finding the minimum spanning tree. You need to specify the graph using a .txt file. Taking an example of a very basic graph .
GitHub - siddharthtumre/Minimum-Spanning-Tree: A java …
A java implementation to find the minimum spanning tree (MST) of a graph using Kruskal's and Prim's algorithms.
Implementing Prim's MST in Java - Stack Overflow
Jul 20, 2019 · I'm having trouble getting my code to follow CLRS's example of a Minimum Spanning Tree (MST) in page 635. I'm also implementing CLRS's pseudo-code for MST-Prim quite literally, which is. In particular, I'm implementing the following graph using an adjacency list: So I actually have two issues.
Implementation of Prim’s Minimum Cost Spanning Tree algorithm (MST …
Implementation of Prim’s Minimum Cost Spanning Tree algorithm (MST), using a simple data structure, as well as Fibonacci heap, and comparison of the relative performance of the two implementations.
PrimMST.java - Princeton University
The {@code weight ()} method returns the * weight of a minimum spanning tree and the {@code edges ()} method * returns its edges. * <p> * This implementation uses <em>Prim's …
Algorithms, 4th Edition by Robert Sedgewick and Kevin Wayne
* @return the edges in a minimum spanning tree (or forest) as * an iterable of edges */ public Iterable edges() { Queue mst = new Queue (); for (int v = 0; v edgeTo.length; v++) { Edge e = edgeTo[v]; if (e != null) { mst.enqueue(e); } } return mst; } /** * Returns the sum of the edge weights in a minimum spanning tree (or forest).