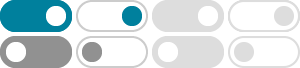
How to Convert Object to Array in JavaScript? - GeeksforGeeks
Aug 30, 2024 · Below are the following approaches to converting Objects to Arrays in JavaScript. The Object.keys () method returns an array of a given object's enumerable property names. Object.keys (obj) takes an object obj as an argument and returns an array containing the keys of the object. The values of the object are included in the resulting array.
javascript - How to convert Set to Array? - Stack Overflow
Create an array from values in a Set: const myArray = [...mySet]; Transform values in a Set using map method to an array: const myArray = [...mySet].map((x) => x * 2); After transforming with map method, maybe you want to store them back in a Set to use the newer Set instance methods like intersection() or difference() etc:
JavaScript Arrays - W3Schools
JavaScript has a built-in array constructor new Array(). But you can safely use [] instead. These two different statements both create a new empty array named points:
JavaScript – Convert String to Array - GeeksforGeeks
Apr 28, 2025 · The Array.prototype.map() method is a powerful tool in JavaScript for transforming or modifying the elements of an array. While map() works directly on arrays, we can also use it to map over a string by first converting the string into an array.
javascript - How to replace item in array? - Stack Overflow
May 7, 2011 · To get every instance use Array.map(): Of course, that creates a new array. If you want to do it in place, use Array.forEach(): For anyone else reading this. Both map () and forEach () are newer additions to the Javascript spec and aren't present in some older browsers.
How to Manipulate Arrays in JavaScript - freeCodeCamp.org
Jun 13, 2019 · splice() changes an array, by adding, removing and inserting elements. slice() copies a given part of an array and returns that copied part as a new array. It does not change the original array. split() divides a string into substrings and returns them as an array.
6 Ways to Convert a String to an Array in JavaScript
Sep 24, 2022 · Converting from string to array is always done using the split() method but after ES6, there are many tools we could do the same. Let’s go through each method one by one and discuss the pros and cons of each.
How to convert a collection to an array in javascript
Mar 1, 2011 · You can use Array.from as it creates a new, shallow-copied Array instance from an iterable. So An HTMLCollection is a collection of document elements. So we can use Array.from to convert to an array them using for each to iterate over its elements of the array.
How to Convert a String to an Array in JavaScript - Stack Abuse
May 22, 2023 · In this tutorial - learn how to convert a string into an array with split(), Regular Expressions (RegEx), the Spread Operator, Array.from() and Object.assign() in JavaScript!
7 ways to convert Objects into Array in JavaScript
Feb 1, 2023 · To convert a JavaScript object into an array is by using the Array.from() method. The Array.from() method creates a new, shallow-copied Array instance from an array-like or iterable object. Here is how
- Some results have been removed