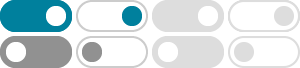
How to change a css class style through Javascript?
The first example will add the class to all DIV elements on the page. The second example will add the new class to all elements that currently have the old class.
Change a Style of all Elements with a specific Class in JS
Mar 5, 2024 · To change the styles of all elements with a specific class: Use the querySelectorAll() method to get a collection of the elements with the specific class. Use the forEach() method to iterate over the collection.
Change the style of an entire CSS class using javascript
Feb 6, 2012 · The key is to define extra rules for additional classes and add these classes to the elements rather than to rewrite the rules for a given style rule. JS. function changeCSS() { var selects = document.getElementsByTagName("select"); for(var i =0, il = selects.length;i<il;i++){ selects[i].className += " hidden"; } } CSS
How to apply style to a class in javascript? - Stack Overflow
Dec 31, 2011 · A simple way would be to document.write the style in the html, while the page is being read, after the link elements are defined. <script> document.writeln('<style>div.box{height:'+ document.documentElement.clientHeight+'px;}'+ '<\/style>'); </script> </head> <body...
How to change style/class of an element using JavaScript
May 1, 2023 · Removing a class name from an HTML element using JavaScript is often used to dynamically modify the styling or behavior of an element based on user interactions, events, or other conditions. The operation can be achieved using classList property, that allows you to add, remove, or toggle classes.
How To Change CSS With JavaScript [With Examples] - Alvaro Trigo
Feb 8, 2024 · With JavaScript, we are able to set CSS styles for one or multiple elements in the DOM, modify them, remove them or even change the whole stylesheet for all your page. Let’s get into the different ways we can do achieve this: 1. Change CSS inline properties with JavaScript.
How to Add Styles to an Element - JavaScript Tutorial
To add inline styles to an element, you follow these steps: First, select the element by using DOM methods such as document.querySelector() . The selected element has the style property that allows you to set the various styles to the element.
How to add, remove, and toggle CSS classes in JavaScript
Mar 4, 2020 · In this article, you'll learn how to add, remove, and toggle CSS classes in JavaScript without jQuery. The simplest way to get and set CSS classes in JavaScript is using the className property. It refers to the value of the HTML element's class attribute. Let us say we have the following HTML element:
Setting CSS Styles using JavaScript - KIRUPA
We can use JavaScript to directly set a style on an element, and we can also use JavaScript to add or remove class values on elements which will alter which style rules get applied. In this tutorial, we're going to learn about both of these approaches.
Change the style of multiple elements with the same class
Jun 18, 2017 · Just replace class_name for the class you want to select. If you just need to change one style you can compact the whole thing to just two lines: var elements = document.getElementsByClassName('class_name'); for (let element of elements) { element.style.display = "none"; }