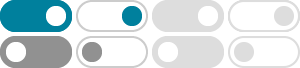
java - How to add new elements to an array? - Stack Overflow
May 16, 2010 · There are many ways to add an element to an array. You can use a temp List to manage the element and then convert it back to Array or you can use the java.util.Arrays.copyOf and combine it with generics for better results. This example will show you how:
add an element to int [] array in java - Stack Overflow
Apr 9, 2013 · Want to add or append elements to existing array int[] series = {4,2}; now i want to update the series dynamically with new values i send.. like if i send 3 update series as int[] series = {4,2,...
java - How to add an element at the end of an array ... - Stack …
As many others pointed out if you are trying to add a new element at the end of list then something like, array[array.length-1]=x; should do. But this will replace the existing element. For something like continuous addition to the array.
Simplest way to add an item to beginning of an array in Java
Feb 2, 2022 · Basically it uses java.util.Arrays.asList() to turn the array into a List, adds to the list and then turns the list back into an array with List.toArray(). As everybody has said, you can't really add to the beginning of an array unless you shift all the elements by one first, or as in my solution, you make a new array.
Adding to an ArrayList Java - Stack Overflow
Oct 28, 2011 · I am a beginner to java, and need some help. I am trying to convert an Abstract Data type Foo which is an associated list to an Arraylist of the strings B. How do you loop through the list and add each string to the array. I may be over thinking it, but I am lost now. Thanks for the help in advance.
java - How can I add new item to the String array? - Stack Overflow
Jan 25, 2013 · A Java array has a fixed length. If you need a resizable array, use a java.util.ArrayList<String> . BTW, your code is invalid: you don't initialize the array before using it.
java - How to add a value to a specified index of array - Stack …
Sep 27, 2013 · Array indexing start from 0, So to insert in 4th place you have to do array[3] = value. So, you'll have to put position = 3. int position = 3; // array index start from 0 Also, since you're using an array, while adding, you'll replace it. If you want to add without replacement, use ArrayList. Refer to this ArrayList Examples if you're ...
How can I dynamically add items to a Java array?
In 99% of cases (more like 99.99999% of cases) if you actually need a primitive array, you're doing something wrong, and it should be handled with OO principles. Unless you're interfacing with native code, or something like Oracle ARRAY objects or some silliness like that, you're much better off avoiding primitive arrays. –
java - Add an object to an Array of a custom class - Stack Overflow
May 1, 2012 · Im a beginner to Java and I'm trying to create an array of a custom class. Let say I have a class called car and I want to create an array of cars called Garage. How can I add each car to the garage? This is what I've got: car redCar = new Car("Red"); car Garage [] = new Car [100]; Garage[0] = redCar;
Java ArrayList how to add elements at the beginning
Jun 18, 2020 · Since you want to add new element, and remove the old one. You can add at the end, and remove from the beginning. That will not make much of a difference. Queue has methods add(e) and remove() which adds at the end the new element, and removes from the beginning the old element, respectively.