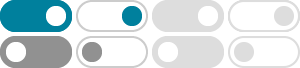
Reading a file using a relative path in a Python project
But there is an often used trick to build an absolute path from current script: use its __file__ special attribute: test = list(csv.reader(f)) This requires python 3.4+ (for the pathlib module). If you still need to support older versions, you can get the same result with: test = list(csv.reader(f))
Open file in a relative location in Python - Stack Overflow
Aug 24, 2011 · from pathlib import Path data_folder = Path("/relative/path") file_to_open = data_folder / "file.pdf" f = open(file_to_open) print(f.read()) Python 3.4 introduced a new standard library for dealing with files and paths called pathlib.
python - How can I create a full path to a file from parts (e.g. path ...
Thus the correct form of your code snippet is: os.path.join(dir_name, base_filename + os.extsep + extension) In Python 3.4 and above, the pathlib standard library module can be used like so:
File and directory Paths - Python Cheatsheet
There are two ways to specify a file path. There are also the dot (.) and dot-dot (..) folders. These are not real folders, but special names that can be used in a path. A single period (“dot”) for a folder name is shorthand for “this directory.” Two periods (“dot-dot”) means “the parent folder.” To see if a path is an absolute path:
Python Import from File – Importing Local Files in Python
Jul 6, 2023 · To import local files in Python using the Pandas library, we can follow these steps: Specify the file path: Determine the file path of the local file we want to import. It can be an absolute path (for example, " C:/path/to/file.csv ") or a relative path (for example, " data/file.csv ").
How to Read from a File in Python - GeeksforGeeks
Mar 13, 2025 · Reading from a file in Python means accessing and retrieving the contents of a file, whether it be text, binary data or a specific data format like CSV or JSON. Python provides built-in functions and methods for reading a file in python efficiently. Example File: geeks.txt.
Python Write to File – Open, Read, Append, and Other File …
May 7, 2020 · We usually use a relative path, which indicates where the file is located relative to the location of the script (Python file) that is calling the open() function. For example, the path in this function call: Only contains the name of the file.
Reading and Writing Files in Python (Guide) – Real Python
In order to access the file, you need to go through the path folder and then the to folder, finally arriving at the cats.gif file. The Folder Path is path/to/. The File Name is cats. The File Extension is .gif. So the full path is path/to/cats.gif.
Python Pathlib: File System Operations in Python
# Joining paths file_path = os.path.join('data', 'processed', 'results.csv') # Getting file name file_name = os.path.basename(file_path) # Checking if a file exists if os.path.exists(file_path) and os.path.isfile(file_path): # Reading a file with open(file_path, 'r') as f: content = f.read()
Python 3 Quick Tip: The easy way to deal with file paths on
Jan 31, 2018 · Python 3.4 introduced a new standard library for dealing with files and paths called pathlib — and it’s great! To use it, you just pass a path or filename into a new Path () object using forward...
- Some results have been removed