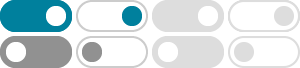
React Components - W3Schools
Example. Create a Class component called Car. class Car extends React.Component { render() { return <h2>Hi, I am a Car!</h2>; } }
React Tutorial - W3Schools
Learning by Examples. Our "Show React" tool makes it easy to demonstrate React. It shows both the code and the result.
React Class Components - W3Schools
Before React 16.8, Class components were the only way to track state and lifecycle on a React component. Function components were considered "state-less". With the addition of Hooks, Function components are now almost equivalent to Class components.
React Forms - W3Schools
In React, form data is usually handled by the components. When the data is handled by the components, all the data is stored in the component state. You can control changes by adding event handlers in the onChange attribute.
React useContext Hook - W3Schools
React Context is a way to manage state globally. It can be used together with the useState Hook to share state between deeply nested components more easily than with useState alone.
React JSX - W3Schools
Example 1. JSX: const myElement = <h1>I Love JSX!</h1>; const root = ReactDOM.createRoot(document.getElementById('root')); root.render(myElement); Run Example »
React Props - W3Schools
React Props. React Props are like function arguments in JavaScript and attributes in HTML. To send props into a component, use the same syntax as HTML attributes:
React useCallback Hook - W3Schools
One reason to use useCallback is to prevent a component from re-rendering unless its props have changed. In this example, you might think that the Todos component will not re-render unless the todos change:
React Conditional Rendering - W3Schools
In React, you can conditionally render components. There are several ways to do this.
React Hooks - W3Schools
Hooks allow function components to have access to state and other React features. Because of this, class components are generally no longer needed. Although Hooks generally replace class components, there are no plans to remove classes from React.