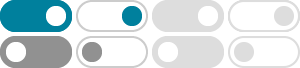
Binary Search Tree - GeeksforGeeks
Feb 8, 2025 · A Binary Search Tree (or BST) is a data structure used in computer science for organizing and storing data in a sorted manner. Each node in a Binary Search Tree has at most two children, a left child and a right child, with the left child containing values less than the parent node and the right child containing values greater than the parent node.
Binary Search Tree - Programiz
A binary search tree is a data structure that quickly allows us to maintain a sorted list of numbers. Also, you will find working examples of Binary Search Tree in C, C++, Java, and Python.
12. Binary search tree – Data Structures and Algorithms
Binary search tree is a data structure that maintains a set of elements. The basic operations are the same as with hashing: elements can be added, searched and removed efficiently. Binary search tree differs from hashing in that it maintains the elements in order.
@Override /** Delete an element from the binary tree. The hasNext() method checks whether current is still in the range of list. element and moves current to point to the next element in the list. element from the tree and a new list is created -current does not need to be changed. @Override /** Obtain an iterator. Use inorder. */
Binary Search Tree Data Structure Explained with Examples
Aug 16, 2024 · A binary search tree (BST) is a rooted binary tree data structure used to store data in a way that allows quick lookup, addition, and removal of items. The key idea is that each node‘s value is greater than all the values in its left subtree, but smaller than the values in …
Binary Search Tree - Online Tutorials Library
Following are the basic operations of a Binary Search Tree −. Search − Searches an element in a tree. Insert − Inserts an element in a tree. Pre-order Traversal − Traverses a tree in a pre-order manner. In-order Traversal − Traverses a tree in an in-order manner. Post-order Traversal − Traverses a tree in a post-order manner.
Binary Search Trees · Data Structures
A binary search tree (BST) is a simple way to implement an ordered dictionary. In fact, the STL (multi)set and (multi)map use a variation of a BST (a red-black tree) as their internal data structure.
Search Trees - CSE 373
Binary search trees are commonly used to implement sets or maps. A collection of unique elements or “keys”. Unlike lists and deques, sets do not maintain indices for each element, which enables more efficient data structure implementations. A collection that associates each unique key with a value.
Mastering Binary Search Trees: Key Concepts and Operations
A Binary Search Tree (BST) is a specialized type of binary tree that organizes data in a hierarchical structure. Each node in a BST contains three elements: a value, a reference to the left child, and a reference to the right child.
12.11. Binary Search Trees — OpenDSA Data Structures and …
Method find takes the search key as an explicit parameter and its BST as an implicit parameter, and returns the record that matches the key. However, the find operation is most easily implemented as a recursive function whose parameters …