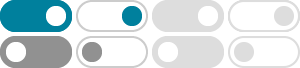
Introduction to Circular Linked List - GeeksforGeeks
Feb 24, 2025 · In this tutorial, we’ll cover the basics of circular linked lists, how to work with them, their advantages and disadvantages, and their applications. What is a Circular Linked List? A circular linked list is a special type of linked list where all the nodes are connected to form a circle.
Circular Linked List Algorithm - Online Tutorials Library
Circular Linked List is a variation of Linked list in which the first element points to the last element and the last element points to the first element. Both Singly Linked List and Doubly Linked List can be made into a circular linked list. In singly linked list, …
Circular Linked List - Programiz
Let's see how we can represent a circular linked list on an algorithm/code. Suppose we have a linked list: Here, the single node is represented as. struct Node * next; . Each struct node has a data item and a pointer to the next struct node. Now we will create a simple circular linked list with three items to understand how this works.
Circular Linked List in C++ - GeeksforGeeks
Jun 24, 2024 · In this article, we will learn how to implement a circular linked list in C++. In C++, to create a circular linked list, we need to define a class name Node that will consist of the data members for a node of the list. After that, we can create a CircularLinkedList class to encapsulate the data members and functions for the circular linked list.
Insertion in Circular Singly Linked List - GeeksforGeeks
Feb 24, 2025 · In this article, we will learn how to insert a node into a circular linked list. Insertion is a fundamental operation in linked lists that involves adding a new node to the list. In a circular linked list, the last node connects back to the first node, creating a …
Data Structures Tutorials - Circular Linked List with an example ...
In data structures, a circular linked list is a linked list in which the last node stores the address of the first node. A circular linked list is a sequence of elements in which every element has a link to its next element in the sequence and the last element has a link to the first element.
Demystifying Algorithms: Circular Linked Lists - DEV Community
Nov 29, 2024 · What is a Circular Linked List? A circular linked list is a variation of a linked list where: The last node points back to the first node, forming a loop. Traversal can continue indefinitely because there’s no null at the end. Circular linked lists can be: Singly Circular: Each node has one pointer (Next), and the last node points to the head.
C program to create and traverse Circular linked list
Nov 6, 2015 · Write a C program to create a circular linked list of n nodes and traverse the list. How to create a circular linked list of n nodes and display all elements of the list in C. Algorithm to create and traverse a Circular linked list. Basic C programming, Function, Dynamic memory allocation, Circular linked list. Being: . alloc (head) read (data)
Circular Linked List | Free Data Structures Course - Talent Battle
Aug 24, 2024 · A circular linked list is a variation of the linked list where the last node points back to the first node, creating a circular structure. This configuration is useful in applications where the entire list needs to be looped through continuously, such as …
Circular Singly Linked List – All c programming & algorithm
Write a program to create a circular linked list. Perform insertion and deletion at the beginning and end of the list. In a circular linked list, the last node contains a pointer to the first node of the list. We can have a circular singly linked list as well as a circular doubly linked list.