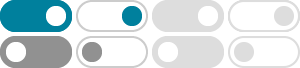
Password validation in Python - GeeksforGeeks
Dec 30, 2022 · This code uses a function that checks if a given password satisfies certain conditions. It uses a single for loop to iterate through the characters in the password string, and checks if the password contains at least one digit, one uppercase letter, one lowercase letter, and one special symbol from a predefined list and based on ascii values.
How do I make a password program in Python 3.4? [closed]
Nov 15, 2014 · Right now your checking for a correct password and user with: Without quotes python expects that bob and rainbow123 are defined variables. Since they're not defined it throws a NameError. Just enclose those values in quotes: #Must Access this to continue. while True: UserName = input ("Enter Username: ") PassWord = input ("Enter Password: ")
Password Authentication With Python: A Step-by-Step Guide
Apr 11, 2022 · This article will show you how to do password authentication with Python. Password Authentication Code: import getpass database = { "Alain" : "123456" , "Sandra" : "654321" } username = input ( "Enter Your Username : " ) password = getpass . getpass ( "Enter Your Password : " ) for i in database . keys ( ) : if username == i : while password ...
Storing and Retrieving Passwords Securely in Python
Dec 29, 2023 · In this comprehensive guide, we’ll cover Python’s best practices for securely saving passwords to a database and retrieving them for authentication. Whether you’re building a new application or improving security in an existing system, this guide has you covered. Also read: Random Password Generator in Python | GUI Tkinter
Create a Random Password Generator using Python
Jan 10, 2023 · Here we will create a random password using Python code. Example of a weak password : password123. Example of a strong password : &gj5hj&*178a1. string – For accessing string constants. The ones we would need are –.
Python program to check the validity of a Password
Sep 6, 2024 · In this program, we will be taking a password as a combination of alphanumeric characters along with special characters, and checking whether the password is valid or not with the help of a few conditions.
Password Authentication using Python | Aman Kharwal
May 2, 2021 · To create a password authentication system using Python you have to follow the steps mentioned below: Create a dictionary of usernames with their passwords. Then you have to ask for user input as the username by using the input function in Python. Then you have to use the getpass module in Python to ask for user input as the password.
Secure Password Handling in Python | Martin Heinz - Medium
Oct 17, 2021 · Let's start simple - you have basic Python application with command line interface. You need to ask user for password. You could use input(), but that would show the password in terminal, to avoid that you should use getpass instead: # Do Stuff...
How to Validate Passwords in Python? - Python Guides
Jan 9, 2025 · One effective way to validate passwords in Python is by using regular expressions (regex). Regex allows you to define a pattern that the password must match. Here’s an example: pattern = "^(?=.*?[A-Z])(?=.*?[a-z])(?=.*?[0-9])(?=.*?[#?!@$%^&*-]).{8,}$" return re.match(pattern, password) is not None. print(validate_password("Passw0rd!")) Output:
Python Program For Password Generator (With Complete Code) - Python …
To create a password generator in Python, you can follow these steps: Import the necessary modules: random and string. Define a function that takes the desired password length as input. Create a string containing all possible characters for the password. This can include uppercase letters, lowercase letters, digits, and special characters.
- Some results have been removed