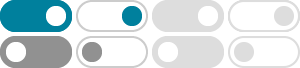
C Program to Reverse a Number
This program takes integer input from the user. Then the while loop is used until n != 0 is false (0). In each iteration of the loop, the remainder when n is divided by 10 is calculated and the value of n is reduced by 10 times. Inside the loop, the reversed number is computed using: reverse = reverse * 10 + remainder;
Reverse Number Program in C - GeeksforGeeks
Jul 17, 2023 · In this article, we will learn how to reverse the digits of a number in C programming language. For example, if number num = 12548, the reverse of number num is 84521. (a) Multiply rev_num by 10 and add remainder of num. divide by 10 to rev_num. rev_num = rev_num*10 + num%10; . (b) Divide num by 10.
Reverse a Number in C using While Loop - StackHowTo
Nov 7, 2021 · We use the modulo operator (%) in the program to get the digits of a number. There are four ways to reverse a number in C, by using for loop, while loop, recursion, or by creating a function. Output:
Reverse of a Number using while loop in C - Coding Connect
Jan 20, 2015 · Program to find reverse of a number in C is used to reverse a given number using while loop and print the reversed number on the output screen.
C Program to Reverse A Number Using Different Methods
Apr 12, 2025 · C Program to Reverse a Number Using While Loop. The first method that we will use to reverse a number in C is through a while loop. We will create and use the while loop to continue processing the formula until the value of the number becomes 0. Here’s the implementation of the program using the while loop. Example. #include <stdio.h> int main()
How to Reverse a Number using Loops in C - Tutorial Kart
In this tutorial, we learned different ways to reverse a number in C: while Loop: Extracts and appends digits iteratively. for Loop: Achieves the same result with a compact loop structure. Recursion: Calls a function repeatedly to reverse digits.
C Program to Reverse a Given Number using While loop, Do-while…
Oct 3, 2024 · In this article, you will learn how to make the reverse of a given number by using programming loops like while, do-while, and for. EXAMPLE: 1234 ==> 4321 Example
C Program to Reverse a Number - Tutorial Gateway
This article discloses how to write a C Program to Reverse a Number using the While Loop, for loop, Functions & Recursion with examples.
C Program to find the reverse of a number - CodinGeek
Feb 28, 2021 · In this C Programming example, we discuss how to find the reverse of an input number using recursion and while loop and discussed the execution of the program.
C Program To Reverse A Number Using While Loop
We are going to write a c program to take a number as an input and reverse that number. We can do this by reversing each single digits of the whole number. long int a,rev,d; clrscr(); printf("\n **************************"); printf("\n Enter Any Number: "); scanf("%ld",&a); rev=0; while(a!=0) d=a%10; rev=rev*10+d; a=a/10;