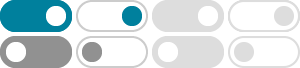
C Program to reverse a given number - BeginnersBook
May 19, 2024 · In this article, we will learn how to write a C program to reverse a number. For example, if the given number is 1234 then program should return number 4321 as output. We will see two different ways to accomplish this. Example …
C program to find reverse of a number using recursion
Mar 7, 2016 · Step by step descriptive logic to find reverse of a number. Multiply reverse variable by 10. Find the last digit of the given number. Add last digit just found to reverse. Divide the original number by 10 to remove last digit, which is not needed anymore.
C Program to Reverse Number Using Recursive Function
Question: Write a program in C to find reverse of a given integer number using recursive function (recursion). result = reverse(number, 0); printf("Reverse of %d is %d.", number, result); return 0; } int reverse(int num, int rev) { if(num ==0) return rev; else return reverse (num /10, rev *10 + num %10); } Reverse of 1234 is 4321.
C Program to Reverse a Number
This program takes integer input from the user. Then the while loop is used until n != 0 is false (0). In each iteration of the loop, the remainder when n is divided by 10 is calculated and the value of n is reduced by 10 times. Inside the loop, the reversed number is computed using: reverse = reverse * 10 + remainder;
C Program to Reverse a Number using Recursion - C Examples …
In this example, you will learn to write a C Program to reverse a number using recursion. When the user enters a number, the program calls itself several times recursively and reverse the number and finally, prints the result.
C Program To Reverse A Number | 6 Ways Explained With Examples …
Ways to write a C program to reverse a number: 1. Using While Loop 2. Using For Loop 3. Using Recursion 4. Using Function 5. Using Arrays 6. Negative Number
C Program to find the reverse of a number - CodinGeek
Feb 28, 2021 · In this C Programming example, we will discuss the steps and implement the C program to reverse an input number using recursion and while loop.
C Program to Reverse a Number using Recursion - Sanfoundry
* C program to find the reverse of a number using recursion. */ #include <stdio.h> #include <math.h> int rev (int, int); int main () { int num, result; int length = 0, temp; printf("Enter an integer number to reverse: "); scanf("%d", & num); . temp = num; while (temp != …
C Program to Reverse a Number - Tutorial Gateway
This article discloses how to write a C Program to Reverse a Number using the While Loop, for loop, Functions & Recursion with examples.
C program to find reverse of a number using recursion
Learn how to write a C program to find the reverse of a number using recursion. This guide covers the concept of recursion, a detailed algorithm, and complete code examples. Reversing a number is a common task in programming that involves rearranging the digits of …
- Some results have been removed