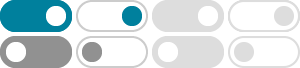
Array of Strings in C++ - GeeksforGeeks
Feb 26, 2025 · The general syntax of array of strings is: string arr_name[size] where arr_name is the name assigned to the array and size is the desired size. Understanding how to create and manage arrays of strings is essential in C++. The C++ Course covers five different methods for creating arrays of strings, helping you choose the right approach for your ...
How to store words in an array in C? - Stack Overflow
Apr 9, 2017 · In C, text strings are stored as arrays of characters. If you are dealing with strings of variable length, you can use pointers to refer to them. Here's how to do it for your example. const char *array1[4]; // use const because we're pointing …
How to declare an array of strings in C++? - Stack Overflow
Jan 28, 2016 · You can directly declare an array of strings like string s[100];. Then if you want to access specific elements, you can get it directly like s[2][90] . For iteration purposes, take the size of string using the s[i].size() function.
how to input array of words c++ - Stack Overflow
Dec 6, 2013 · std::copy_if(std::begin(words), std::end (words), std::ostream_iterator<std::string>(std::cout, " "), [](const std::string& word){ return word.find("stu") == 0; }); This uses a few C++11 features ( copy_if , lambdas, non-member begin / end ).
How to store words in an array in C? - GeeksforGeeks
Oct 18, 2019 · We all know how to store a word or String, how to store characters in an array, etc. This article will help you understand how to store words in an array in C. To store the words, a 2-D char array is required.
Understanding C++ String Array - DigitalOcean
Aug 4, 2022 · C++ provides us with ‘string’ keyword to declare and manipulate data in a String array. The string keyword allocates memory to the elements of the array at dynamic or run-time accordingly. Thus it saves the headache of static memory allocation of data-elements.
Efficient Ways To Work With Arrays Of Strings In C++
May 14, 2024 · Learn how to initialize, access, modify, search, and delete strings in a C++ array efficiently. Improve your coding skills today!
Arrays and Strings in C++ - GeeksforGeeks
May 7, 2020 · The std::string::at() in C++ is a built-in function of std::string class that is used to extract the character from the given index of string. In this article, we will learn how to use string::at() in C++.
Storing words of a string into an array - C++ Forum - C++ Users
Feb 22, 2018 · I've tried placing if statements in several locations, but then it won't store any of the words into the array. struct Word { string english; string piglatin; Word *wordArr = new Word[size]; int position; int j = 0; cout << size << endl; for (unsigned int i = 0; i < words.length(); i++) if (isspace(words[i])) { if (position != '\0') {
C++ Array of Strings - Online Tutorials Library
In this section we will see how to define an array of strings in C++. As we know that in C, there was no strings. We have to create strings using character array.