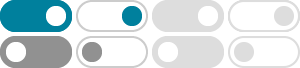
python - How can I add new keys to a dictionary? - Stack Overflow
Add new keys to a nested dictionary. If a key has to be added to a dictionary that is nested inside a dictionary, dict.setdefault (or collections.defaultdict) is really useful. For example, let's try to add a new key-value pair to mydict only nested inside another key: 'address'.
python - How to add key,value pair to dictionary? - Stack Overflow
I got here looking for a way to add a key/value pair(s) as a group - in my case it was the output of a function call, so adding the pair using dictionary[key] = value would require me to know the name of the key(s). In this case, you can use the update method: dictionary.update(function_that_returns_a_dict(*args, **kwargs)))
How to add new values to existing dictionary in python
Sep 23, 2019 · if key in dictionary: dictionary[key].append(message1) else: dictionary[key] = [message1] If key already exists in dictionary , this appends message to dictionary[key] . Otherwise, it creates a new single-item list [message1] as the value of dictionary[key] .
Update python dictionary (add another value to existing key)
Jan 24, 2017 · You can create your dictionary assigning a list to each key. d = {'word': [1], 'word1': [2]} and then use the following synthases to add any value to an existing key or to add a new pair of key-value: d.setdefault(@key,[]).append(@newvalue) For your example will …
python - Iterating over dictionaries using 'for' loops - Stack Overflow
Jul 21, 2010 · will simply loop over the keys in the dictionary, rather than the keys and values. To loop over both key and value you can use the following: For Python 3.x: for key, value in d.items(): For Python 2.x: for key, value in d.iteritems(): To test for yourself, change the word key to poop.
python - How to add multiple values to a dictionary key ... - Stack ...
The magic of setdefault is that it initializes the value for that key if that key is not defined. Now, noting that setdefault returns the value, you can combine these into a single line: a.setdefault("somekey", []).append("bob")
python 3.x - How to add key and value pairs to new empty …
Jan 30, 2021 · In Python, dictionary keys are unique. If you assign a value to the same key again, it gets overwritten. Example : dct = {} dct["key"] = "value" print(dct) dct["key"] = "value2" print(dct) Output : {"key": "value"} {"key": "value2"} The only option you have is to create a list of amounts and a list of ids:
python - Adding item to Dictionary within loop - Stack Overflow
Jul 2, 2015 · In your current code, what Dictionary.update() does is that it updates (update means the value is overwritten from the value for same key in passed in dictionary) the keys in current dictionary with the values from the dictionary passed in as the parameter to it (adding any new key:value pairs if existing) . A single flat dictionary does not ...
python - How to print the value of a specific key from a dictionary ...
If you want to fetch key based on index, in older version of Python's dictionary were unordered in nature. However from Python 3.6, they maintain the insertion order. – Moinuddin Quadri
python - Reverse / invert a dictionary mapping - Stack Overflow
For inverting key-value pairs in your dictionary use a for-loop approach: # Use this code to invert dictionaries that have non-unique values inverted_dict = dict() for key, value in my_dict.items(): inverted_dict.setdefault(value, list()).append(key) Second Solution. Use a dictionary comprehension approach for inversion: