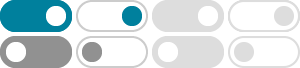
Initializing a C# array with multiple copies of the same element
I want to do a similar thing in C#, more specifically creating an array of n doubles with all elements initialized to the same value x. I have come up with the following one-liner, relying on generic collections and LINQ: double[] v = new double[n].Select(item => x).ToArray();
c# - How do I find duplicates in an array and display how many …
I'm working on a code that prints out duplicated integers from an array with the number of their occurrence. I'm not allowed to use LINQ, just a simple code. I think I'm so close but confused about how to get a correct output: static void Main(string[] args) int[] array = { 10, 5, 10, 2, 2, 3, 4, 5, 5, 6, 7, 8, 9, 11, 12, 12 }; int count = 1;
How to duplicate value of an array n number of times in C#
Jan 22, 2016 · One way is to use Enumerable.Repeat to get n-arrays and SelectMany to flatten them to 1 array with n elements. This works even if the array contains more than one element: AddrArra = Enumerable.Repeat(AddrArra, n).SelectMany(arr => arr).ToArray(); But i would prefer a custom extension method which is readable and more efficient:
Find duplicate elements in an array - GeeksforGeeks
Dec 19, 2024 · The idea is to use a nested loop and for each element check if the element is present in the array more than once or not. If present, then check if it is already added to the result. If not, then add to the result.
Multiple ways to find duplicates in c# array with pros and cons
Apr 21, 2023 · In C#, we can use various approaches to find duplicate elements in array. Each have pros and cons. We will use 3 approaches in this article. Using a HashSet; Using a Dictionary; Using LINQ;...
Find duplicates in an array in C# - Techie Delight
Feb 16, 2022 · This post will discuss how to find all duplicates in an array in C#. 1. Using Enumerable.GroupBy Method. The idea is to group the elements based on their value and then filter the groups that appear more than once. This can be done with LINQ’s Enumerable.GroupBy() method.
Leveraging Enumerable.Repeat in C#: Generating Repeated Elements …
Sep 1, 2023 · The Enumerable.Repeat method in C# simplifies the process of generating sequences of repeated elements or initializing collections with default values. By leveraging this method, you can write more concise and readable code, making your applications more efficient and maintainable.
Create Array with Non-Default Repeated Values in C#
Aug 19, 2020 · We can create an array with non-default values using Enumerable.Repeat(). It repeated a collection with repeated elements in C#. Firstly, set which element you want to repeat and how many times.
How do I check if my array has repeated values inside it?
Console.WriteLine("Contains duplicates"); The GroupBy method will group any identical elements together, and Any return true if any of the groups has more than one element. Both of the above solutions work by utilizing a HashSet<T>, but you can use one directly like this: Console.WriteLine("Contains duplicates");
How to Remove Duplicate Values From an Array in C#?
Nov 4, 2024 · Removing duplicate elements from an array is necessary for data integrity and efficient processing. The approaches implemented and explained below will use the Lodash to remove duplicate elements from an array.
- Some results have been removed