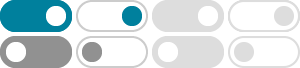
Python Program to find transpose of a matrix - GeeksforGeeks
May 9, 2023 · Transpose of a matrix is obtained by changing rows to columns and columns to rows. In other words, transpose of A[][] is obtained by changing A[i][j] to A[j][i]. For Square Matrix: The below program finds transpose of A[][] and stores the result in B[][], we can change N for different dimension.
Python | Numpy matrix.transpose() - GeeksforGeeks
Jan 9, 2024 · `numpy.matrix.transpose ()` is a function in the NumPy library that computes the transpose of a matrix. It swaps the rows and columns of the matrix, effectively reflecting it along its main diagonal. The function is called on a NumPy matrix object, and it …
Python Program to Transpose a Matrix
Transpose of a matrix is the interchanging of rows and columns. It is denoted as X'. The element at ith row and jth column in X will be placed at jth row and ith column in X'. So if X is a 3x2 matrix, X' will be a 2x3 matrix. Here are a couple of ways to accomplish this in Python.
numpy.matrix.transpose — NumPy v2.2 Manual
numpy.matrix.transpose# method. matrix. transpose (* axes) # Returns a view of the array with axes transposed. Refer to numpy.transpose for full documentation. Parameters: axes None, tuple of ints, or n ints. None or no argument: reverses the order of the axes.
Transpose a matrix in Single line in Python - GeeksforGeeks
Aug 16, 2023 · There are various approaches to find the Transpose of a matrix in a single line in Python. In this article, we will discuss all the approaches which are specific to transposing a matrix in a single line in Python. Using List Comprehension; Using zip; Using NumPy; Using itertools; Transpose Matrix In Single Line using List Comprehension
Transpose Matrix using Python: A Comprehensive Guide
Sep 19, 2023 · Understanding how to transpose a matrix is a foundational skill in linear algebra, and its application in Python is equally significant. This ultimate guide aims to demystify the techniques for reorienting matrices in Python, covering methods from simple one-liners to more complex algorithms.
Matrix Transpose in Python: A Comprehensive Guide
Apr 1, 2025 · This blog will explore the concept of matrix transpose in Python, its usage methods, common practices, and best practices to empower you to handle matrices more effectively in your projects. In the realm of linear algebra and data manipulation, the matrix transpose is a fundamental operation.
5 Best Ways to Find the Transpose of a Matrix in Python
Mar 10, 2024 · This article discusses five different methods to calculate the transpose of a matrix in Python. This method leverages the power of nested list comprehension in Python, which provides an elegant and concise way to create lists.
Transpose Matrix in Python: Concepts, Usage, and Best Practices
Feb 6, 2025 · In Python, working with matrix transposes is a common task in various fields such as data analysis, machine learning, and scientific computing. This blog post will explore how to perform matrix transposes in Python, covering different methods and best practices.
How to Transpose a Matrix in Python: An In-Depth Guide
Nov 14, 2023 · We‘ve covered a comprehensive set of techniques to transpose matrices in Python: numpy.transpose() provides the fastest method optimized for NumPy arrays. Nested loops directly traverse matrix swapping indices. List comprehensions concisely generate transposed elements. zip() efficiently aggregates elements …