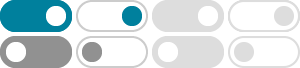
How do I use only numpy to apply filters onto images?
Jul 23, 2020 · To fix this issue, you have to convert the float arrays to np.uint8 and use the 'L' mode in PIL. As for doing convolutions, SciPy provides functions for doing convolutions with kernels that you may find useful. But since we're solely using NumPy, let's implement it!
How to Use NumPy for Basic Image Filtering (3 Techniques)
Jan 23, 2024 · NumPy, a popular Python library for numerical computing, facilitates efficient operations on large arrays and matrices, which can also be applied to images. In this guide, we will explore some basic image filtering techniques using NumPy.
How does one correctly apply filters to an image array?
Oct 27, 2015 · After converting said image to grayscale, I need to apply the following filter float[][] smoothKernel = { {0.1f,0.1f,0.1f}, {0.1f,0.2f,0.1f}, {0.1f,0.1f,0.1f} };
A Demonstration of Filters in Image Processing
Mar 30, 2021 · Filters are operations performed on images. Just as we sliced a portion of the image array to produce certain color effects, filters are produced in like manner. Filters can be used to blur...
Stanford Artificial Intelligence Laboratory
We will examine more closely image filtering. The goal of using filters is to modify or enhance image properties and/or to extract valuable information from the pictures such as edges, corners, and blobs. Here are some examples of what applying filters …
Convolution: Image Filters, CNNs and Examples in Python
Jun 7, 2023 · Convolutions are based on the idea of using a filter, also called a kernel, and iterating through an input image to produce an output image. This story will give a brief explanation of...
Image filtering — Image analysis in Python - scikit-image
For our first example of a filter, consider the following filtering array, which we’ll call a “mean kernel”. For each pixel, a kernel defines which neighboring pixels to consider when filtering, and how much to weight those pixels.
Image Filtering Using Convolution in OpenCV | LearnOpenCV
Jun 7, 2021 · Here, we will explain how to use convolution in OpenCV for image filtering. You will use 2D-convolution kernels and the OpenCV Computer Vision library to apply different blurring and sharpening techniques to an image. We will show you how to implement these techniques, both in Python and C++. How to Use Kernels to Sharpen or Blur Images?
Python and OpenCV: Apply Filters to Images - AskPython
Jun 3, 2021 · Today in this tutorial, we will be applying few of the filters to images. Exciting right? Let’s begin! 1. Importing Modules. The first step is to import the required modules which include OpenCV, matplotlib, and numpy module. We will also change the plotting style to seaborn for better visualization. 2. Loading the initial image.
A Practical Guide to Image Filtering with Python: Kernels Explained
Apr 15, 2024 · # Load the image img = Image.open('eiffel.jpg') # Resize the image to 50x50 pixels img_resized = img.resize((100, 100)) # Convert the resized image to a numpy array image_array = np.array(img_resized)