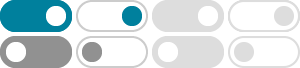
Java Numbers and Strings - W3Schools
If you add a number and a string, the result will be a string concatenation: Example String x = "10"; int y = 20; String z = x + y; // z will be 1020 (a String)
How to add the two or more numbers in to string in java?
Jan 9, 2020 · String + int -> String; You then have the following line: ((((int + int) + String) + int) + int) Resolving the operation one after the other, you end with the following operations to resolve: int(10) + int(10) -> int(20) int(20) + String( Hello ) -> String (20 Hello) String (20 Hello) + int(20) -> String (20 Hello 20)
integer - How to concatenate int values in java? - Stack Overflow
Apr 20, 2010 · int result = a * 10000 + b * 1000 + c * 100 + d * 10 + e; String s = Integer.toString(result); will work. Note: this will only work when a is greater than 0 and all of b, c, d and e are in [0, 9]. For example, if b is 15, Michael's method will get …
java - Why does adding a String and an int act this way
Jul 25, 2012 · What does it mean to "add" a String and an int? In Java, you must have the same type on both sides of an operator to perform the desired action. In many cases when there is a mismatch, Java will convert one value to the same type as the other.
Java Convert int to String – Different Ways of Conversion
Apr 9, 2025 · Ways to Convert int to a String in Java. Converting Integers to Strings involves using the Integer classes toString() or String.valueOf() for direct conversion. String.format() is another method offering flexible formatting options. Using StringBuilder or StringBuffer for appending integer values as strings is efficient for extensive string ...
Concatenate String and Integers in Java - Online Tutorials Library
Learn how to concatenate a string with integers in Java with this comprehensive guide, including examples and code snippets. Discover how to efficiently concatenate strings and integers in Java with practical examples.
String (Java Platform SE 8 ) - Oracle Help Center
public String(int[] codePoints, int offset, int count) Allocates a new String that contains characters from a subarray of the Unicode code point array argument. The offset argument is the index of the first code point of the subarray and the count argument specifies the length of the subarray.
Adding Int To String Java: Adding Integers Made Easy - JA-VA …
Sep 25, 2023 · In Java, you can add integers to strings by leveraging the String.valueOf () method. This method is commonly used to convert primitive data types, including integers, into their string representations. Let’s explore how to use String.valueOf () …
How to convert int to String in Java - CodeGym
Dec 17, 2024 · In this article we are going to discuss converting int (primitive type) and Object type (wrapper) Integer to String. There are many ways to do it in Java. The easiest way to convert int to String is very simple. Just add to int or Integer …
How do I put an int in a string (java) - Stack Overflow
Mar 16, 2011 · Not sure exactly what you are asking for but you can convert int to String via String sequence= Integer.toString(sum); and String to int via int sum = Integer.parseInt(sequence.trim());